Developer Guide
Table of Contents
Product Description
Product Description
CheckMate is a powerful room booking and management system designed for hotel employees, especially those in administrative and management positions. It streamlines the process of room bookings, offering real-time room data visualization, search functionality, and efficient booking management.
Acknowledgements
Acknowledgements
- CheckMate logo generated by: BrandCrowd
- Doughnut Chart inspired by and template taken from user
jewelsea
’s comment in this Stack Overflow post
Setting up, getting started
Setting up, getting started
Refer to the guide Setting up and getting started.
Design
Design

.puml
files used to create diagrams in this document docs/diagrams
folder. Refer to the PlantUML Tutorial at se-edu/guides to learn how to create and edit diagrams.
Architecture
The Architecture Diagram given above explains the high-level design of the App.
Given below is a quick overview of main components and how they interact with each other.
Main components of the architecture
Main
(consisting of classes Main
and MainApp
) is in charge of the app launch and shut down.
- At app launch, it initializes the other components in the correct sequence, and connects them up with each other.
- At shut down, it shuts down the other components and invokes cleanup methods where necessary.
The bulk of the app’s work is done by the following four components:
-
UI
: The UI of the App. -
Logic
: The command executor. -
Model
: Holds the data of the App in memory. -
Storage
: Reads data from, and writes data to, the hard disk.
Commons
represents a collection of classes used by multiple other components.
How the architecture components interact with each other
The Sequence Diagram below shows how the components interact with each other for the scenario where the user issues the command delete 1
.
Each of the four main components (also shown in the diagram above),
- defines its API in an
interface
with the same name as the Component. - implements its functionality using a concrete
{Component Name}Manager
class (which follows the corresponding APIinterface
mentioned in the previous point.
For example, the Logic
component defines its API in the Logic.java
interface and implements its functionality using the LogicManager.java
class which follows the Logic
interface. Other components interact with a given component through its interface rather than the concrete class (reason: to prevent outside component’s being coupled to the implementation of a component), as illustrated in the (partial) class diagram below.
The sections below give more details of each component.
UI component
The API of this component is specified in Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, PersonListPanel
, StatusBarFooter
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class which captures the commonalities between classes that represent parts of the visible GUI.
The UI
component uses the JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
- executes user commands using the
Logic
component. - listens for changes to
Model
data so that the UI can be updated with the modified data. - keeps a reference to the
Logic
component, because theUI
relies on theLogic
to execute commands. - depends on some classes in the
Model
component, as it displaysPerson
object residing in theModel
.
Logic component
API : Logic.java
Here’s a (partial) class diagram of the Logic
component:
The sequence diagram below illustrates the interactions within the Logic
component, taking execute("delete 1")
API call as an example.

DeleteCommandParser
should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram.
How the Logic
component works:
- When
Logic
is called upon to execute a command, it is passed to anBookingBookParser
object which in turn creates a parser that matches the command (e.g.,DeleteCommandParser
) and uses it to parse the command. - This results in a
Command
object (more precisely, an object of one of its subclasses e.g.,DeleteCommand
) which is executed by theLogicManager
. - The command can communicate with the
Model
when it is executed (e.g. to delete a person). - The result of the command execution is encapsulated as a
CommandResult
object which is returned back fromLogic
.
Here are the other classes in Logic
(omitted from the class diagram above) that are used for parsing a user command:
How the parsing works:
- When called upon to parse a user command, the
BookingBookParser
class creates anXYZCommandParser
(XYZ
is a placeholder for the specific command name e.g.,AddCommandParser
) which uses the other classes shown above to parse the user command and create aXYZCommand
object (e.g.,AddCommand
) which theBookingBookParser
returns back as aCommand
object. - All
XYZCommandParser
classes (e.g.,AddCommandParser
,DeleteCommandParser
, …) inherit from theParser
interface so that they can be treated similarly where possible e.g, during testing.
Model component
API : Model.java
The Model
component,
- stores the booking book data i.e., all
Booking
objects (which are contained in aUniqueBookingList
object). - stores the currently ‘selected’
Booking
objects (e.g., results of a search query) as a separate filtered list which is exposed to outsiders as an unmodifiableObservableList<Booking>
that can be ‘observed’ e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. - stores a
UserPref
object that represents the user’s preferences. This is exposed to the outside as aReadOnlyUserPref
objects. - does not depend on any of the other three components (as the
Model
represents data entities of the domain, they should make sense on their own without depending on other components)

RoomTypeTag
which Room
declares. This allows ‘Room’ to have a unique ‘RoomTypeTag’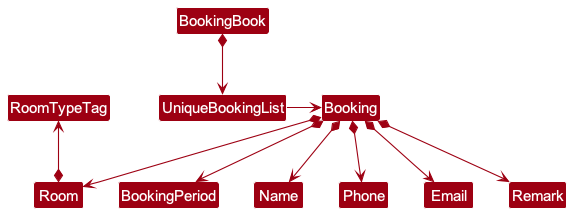
Storage component
API : Storage.java
The Storage
component,
- can save both booking book data and user preference data in JSON format, and read them back into corresponding objects.
- inherits from both
BookingBookStorage
andUserPrefStorage
, which means it can be treated as either one (if only the functionality of only one is needed). - depends on some classes in the
Model
component (because theStorage
component’s job is to save/retrieve objects that belong to theModel
)
Common classes
Classes used by multiple components are in the seedu.addressbook.commons
package.
Implementation
Implementation
This section describes some noteworthy details on how certain features are implemented.
Room Statistics PieChart
Implementation
The Room Statistics PieChart is facilitated by the RoomPieChart class, and the actual pie chart object is created using the DoughnutChart class. This is designed to enable hotel receptionists to be able to determine the number of available and unavailable rooms at a glance during the hustle and bustle of handling multiple guest check ins, allowing them to be able to either report to their manager or supervisor the current number of rooms occupied, or to inform a guest that they are entertaining that perhaps a specific room that they desire is not available due to the large number of rooms occupied on that day.
The primary functions of the class include:
-
RoomPieChart(ObservableList<Booking> bookingList)
- Creates the pie chart object with the appropriate dimensions and labels.
The RoomPieChart object is updated by the handleViewRoomStatistics()
method declared in /main/java/seedu/address/ui/MainWindow.java class,
which is called after every command except find
command is returned. The reason why it is not updated after find
is because
the pie chart is generated using the filteredList returned after every command, and since find
generates a filteredList
based on the user’s input, we do not want to update the pie chart with only the Bookings that fit the user’s input.
The following sequence diagram provides a visual representation of the pie chart being updated after each command result is returned:
Design Considerations:
Aspect: Execution of handleViewRoomStatistics():
-
Choice: Edit the CommandResult method signature to take in another boolean parameter to indicate whether handleViewRoomStatistics is called.
- Pros: For every command, it is easy to edit the command’s class to either update the pie chart or not.
- Cons: Might be too simple of an implementation. If the developer chooses to add in more complex commands that rely on other commands, regressions may occur.
-
Alternative: Use a ListChangeListener object to monitor the list to see if there is a change in the number of bookings, and call handleViewRoomStatistics accordingly.
- Pros: Reduces chances of regressions occuring when more complex commands are added as updating the pie chart only relies on any changes to the Bookings list.
- Cons: Demands a more complex implementation.
Aspect: Indication of room pie chart failures:
-
Choice: Show pie chart
- Pros: Visually noticeable to users as they can see if there is no change to the pie chart when there should be or the pie chart is not shown at all.
- Cons: Users may somehow not notice that the pie chart is not changing.
Prefix Autocomplete
Implementation
The prefix autocomplete mechanism is facilitated by the PrefixCompletion
class. This class is designed to recommend possible prefixes that a user can use to complete their commands more efficiently.
The primary functions of the class include:
-
PrefixCompletion#getNextCompletion(String currentInput)
- Provides the next possible prefix recommendation based on the user’s current input.
PrefixCompletion
is used in the CommandBox
class. When the user presses the TAB
key, the handleTabPressed
method is invoked, which runs the PrefixCompletion
to auto-complete the user’s current input.
Given below is an example usage scenario and how the prefix completion mechanism behaves at each step.
- The user starts typing a command in the command box.
- Upon pressing the
TAB
key, the current text in the command box is passed to thePrefixCompletion#getNextCompletion
method. - The method checks the user’s input to determine the appropriate command (e.g.,
add
,edit
) and use necessaryPrefixFinder
to find the unused prefix. - If a suitable prefix is identified, it is appended to the user’s current input in the command box, accompanied by an example value. The example value is highlighted for easy replacement by the user.
- If no suitable prefix is identified or if the user’s input isn’t recognized, the command box runs
CommandBox#indicateCompletionFailure()
which signals the user to adjust their input by adjusting the text color for 0.5s.
The following sequence diagram provides a visual representation of the prefix autocomplete operation within the CommandBox
:
Design Considerations:
Aspect: Execution of prefix autocomplete:
-
Choice: Uses a predefined list of prefixes and examples for each command.
- Pros: Simplified implementation. Easy to maintain and update.
- Cons: -Limited to predefined prefixes and commands. -Subject to manual change when prefix changes.
-
Alternative: Dynamically generate example suggestions based on user’s history or frequently used parameter.
- Pros: Offers more personalized and relevant suggestions.
- Cons: Demands a more complex implementation. Requires a mechanism to track and evaluate user’s command history.
Aspect: Indication of prefix autocomplete failures:
-
Choice: Flash the command box with an error style briefly.
- Pros: Visually noticeable. Informs the user that the autocomplete attempt was unsuccessful.
- Cons: Might be considered intrusive by some users.
-
Alternative: Display a tooltip or a message below the command box.
- Pros: Less intrusive. Clearly communicates the message to the user.
- Cons: Potential to clutter the UI if not managed gracefully.
RoomType Tags
Implementation
The Room class uses the RoomType enumeration to categorize rooms by type and employs the RoomTypeTag class to generate corresponding UI labels. The RoomType enumeration inside the Room class is equipped with a static mapping from room numbers to their associated types, enabling swift identification of a room’s category based on its number. This categorization is then visually represented in the UI by creating RoomTypeTag objects that serve as labels for each room.
The primary functions of the class include:
-
RoomType getRoomTypeByNumber(int roomNumber)
- Uses an internal map to associate room numbers with specific room types, thus enabling quick determination of a room’s type.
Given below is an example usage scenario and how the prefix completion mechanism behaves at each step.
- The user enters the
add
command with the room number. - A Room object is instantiated with a room number passed as a string to the constructor.
- The constructor parses the string into an integer and calls RoomType.getRoomTypeByNumber to ascertain the room’s category.
- A RoomTypeTag object is created using the name of the determined RoomType, which then facilitates the creation of a UI label for display.
The following sequence diagram provides a visual representation of the prefix autocomplete operation within the CommandBox
:
Design Considerations:
Aspect: Management of room types:
-
Choice: Utilize the RoomType enumeration within the Room class.
- Pros: Ensures clear and centralized room type definitions, simplifying updates and maintenance.
- Cons: -The static nature of enumeration can limit flexibility, making it harder to adapt to dynamic changes. -The direct association between room numbers and types might not easily support modifications in room categorization.
-
Alternative: Implement a separate RoomType class with dynamic properties.
- Pros: Adds the ability to handle dynamic changes and offers potential for future scalability.
- Cons: Complicates the implementation, possibly necessitating new mechanisms for maintaining and retrieving room type information.
Aspect: Representation of room types as UI labels:
-
Choice: Create RoomTypeTag objects from the names of RoomType enumeration constants.
- Pros: This direct conversion guarantees that the data model aligns seamlessly with the UI’s visual elements.
- Cons: -Utilizes the RoomTypeTag class, which may have been initially intended for a broader tagging purpose. -Could lead to unnecessary complexity if the RoomTypeTag class functionality significantly overlaps with the RoomType enumeration.
-
Alternative: Design a specialized UI component dedicated to room types.
- Pros: Enables custom behavior and appearance specific to room type representation.
- Cons: Requires additional development work and may result in code redundancy if not carefully integrated with the existing UI components.
JSON Injection Parser
Implementation
The JSON Injection Parser is facilitated by the JsonInjectionParser
class. It is simply the first parser to be used when parsing through the user input, meant to prevent the user
from inputting possible JSON commands that may be executed when dealing with storing or retrieving memory from the JSON file.
The primary functions of the class include:
-
parse(String args) throws ParseException
- Parses through the user input to check if the input contains any escape characters or characters that could be used to form a JSON command, and throws a ParseException if the input does contain any of the banned characters.
The following sequence diagram provides a visual representation of the JsonInjectionParser.parse() method being used after a command is passed to Logic.
Design Considerations:
Aspect: Execution of parse():
-
Choice: Prevent the user from inputting any characters that may constitute a JSON command.
- Pros: Simple to implement.
- Cons: This may cause inconvenience to the user.
-
Alternative: Sanitise the input, ie: remove the banned characters from the input and execute the command.
- Pros: Less inconvenience to the user.
- Cons:
- More complex implementation.
- Due to the input having some characters removed, the command may either may no sense at all, or in successful execution the details of the command may not make sense to the user at all.
Appendix: Requirements
Appendix: Requirements
Product scope
Target User profile
- Hotel employees, especially those in administrative and management roles.
- Has a need to manage a significant number of bookings in a hotel.
- Prefer desktop apps over other types
- Can type fast
- Prefers typing to mouse interactions
- Is reasonably comfortable using CLI apps
Value Proposition
CheckMate empowers hotel employees to efficiently manage room bookings, optimize room-booking matching, and enhance guest experiences. It offers real-time room availability, service scheduling, and amenity management. It is optimized for administrative roles, ensuring seamless room allocation and guest service.
User stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can… |
---|---|---|---|
* * * |
hotel employee | add a new room booking | keep track of who is staying in which room |
* * * |
hotel employee | cancel an existing room booking | manage changes in client plans or circumstances |
* * * |
hotel employee | edit details of an existing booking | make changes as required |
* * * |
hotel employee | view a list of all bookings | quickly understand room occupancy |
* * * |
hotel employee | search for a booking using the client’s name | quickly retrieve their details |
* * * |
hotel employee | view room availability | manage and plan room allocations effectively |
* * * |
hotel employee | view a client’s check-in/check-out times | keep records for management and service improvement |
* * |
hotel employee | assign a specific room number to a client | ensure clients know where to stay |
* * |
hotel employee | reassign a different room number to a client | accommodate changes or needs |
* * |
hotel employee | leave notes on a booking | note special requests or important information |
* * |
hotel employee | handle special requests | provide personalized service to clients |
* * |
admin | set different room categories | offer diverse accommodation options |
* * |
hotel employee | check out a client from their room | manage room availability at the end of stays |
* |
admin | set room rates | ensure clients are charged appropriately |
* |
hotel employee | apply discounts to a booking | provide promotions and special offers to clients |
* |
hotel employee | handle client complaints | ensure customer satisfaction and address issues |
* |
hotel employee | schedule room cleaning | maintain cleanliness and readiness of rooms |
* |
hotel employee | block rooms for maintenance | ensure no bookings are made for unavailable rooms |
* |
hotel employee | view the history of a room | track and understand room usage over time |
* |
hotel employee | process payments for room bookings | manage financial transactions for stays |
* |
hotel employee | issue refunds | manage cancellations and returns appropriately |
* |
admin | generate daily reports | understand hotel operations and finances |
* |
admin | set schedules for hotel employees | ensure adequate staffing for hotel operations |
* |
admin | add new rooms to the hotel’s inventory | expand and update the hotel’s room offerings |
* |
hotel employee | archive old bookings | keep the system organized and up-to-date |
* |
admin | set up promotional offers | attract clients and offer competitive services |
* |
hotel employee | manage online bookings | handle reservations made through digital platforms |
* |
hotel employee | send booking confirmation emails | inform clients about their booking status |
* |
hotel employee | handle emergency situations | ensure safety and rapid response in crises |
* |
admin | manage the hotel’s inventory | maintain adequate supplies of necessary items |
* |
hotel employee | manage group bookings | accommodate events or large groups effectively |
* |
admin | monitor hotel security | ensure safety and security within the hotel premises |
* |
hotel employee | receive and manage client feedback | improve services based on client suggestions |
* |
hotel employee | coordinate with other departments | provide seamless service across the hotel |
* |
hotel employee | manage lost and found items | assist clients with their misplaced belongings |
* |
hotel employee | manage long-term bookings | cater to clients staying for extended periods |
* |
hotel employee | organize transport for clients | provide additional services for client convenience |
Use cases
(For all use cases below, the System is the CheckMate
and the Actor is the user
, unless specified otherwise)
Use case: Delete a person
MSS
- Hotel employee requests to add a booking.
- CheckMate prompts for booking details.
- Hotel employee enters the booking details.
-
CheckMate adds the booking and confirms the addition.
Use case ends.
Extensions:
- 3a. The entered details are invalid.
- 3a1. CheckMate shows an error message.
- 3a2. Hotel employee re-enters the details.
Use case resumes at step 3.
Use case: Cancel a booking
MSS:
- Hotel employee requests to list all bookings.
- CheckMate displays the list of bookings.
- Hotel employee selects a booking to cancel.
-
CheckMate cancels the selected booking and confirms the cancellation.
Use case ends.
Extensions:
-
2a. The booking list is empty.
Use case ends.
-
3a. The selected booking index is invalid.
- 3a1. CheckMate shows an error message.
Use case resumes at step 2.
Use case: Edit a booking
MSS:
- Hotel employee requests to edit a booking.
- CheckMate prompts for the booking index and new details.
- Hotel employee enters the booking index and new details.
-
CheckMate updates the booking and confirms the changes.
Use case ends.
Extensions:
- 3a. The provided index or details are invalid.
- 3a1. CheckMate displays an error message.
Use case resumes at step 2.
Use case: View all bookings
MSS:
- Hotel employee requests to view all bookings.
-
CheckMate displays a list of all bookings.
Use case ends.
Use case: Search for a booking
MSS:
- Hotel employee requests to search for a booking.
- CheckMate prompts for search criteria.
- Hotel employee enters search criteria.
-
CheckMate displays bookings matching the criteria.
Use case ends.
Extensions:
- 3a. No bookings match the search criteria.
- 3a1. CheckMate shows a message that no matches were found.
Use case resumes at step 2.
Non-Functional Requirements
- Should work on any mainstream OS as long as it has Java
11
or above installed. - Should be able to hold up to 1000 bookings without a noticeable sluggishness in performance for typical usage.
- A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
- Should have a user-friendly and intuitive graphical user interface (GUI) that complements the command line interface (CLI), making it accessible for users who may not be as comfortable with CLI.
- Response time for any command should not exceed 2 seconds, ensuring quick feedback and a smooth user experience.
Glossary
- Mainstream OS: Windows, Linux, Unix, OS-X
- Private contact detail: A contact detail that is not meant to be shared with others
Appendix: Instructions for manual testing
Appendix: Instructions for manual testing
Given below are instructions to test the app manually.

Launch and shutdown
- Initial launch
- Download the jar file and copy into an empty folder
- Double-click the jar file Expected: Shows the GUI with a set of sample contacts. The window size may not be optimum.
- Saving window preferences
- Resize the window to an optimum size. Move the window to a different location. Close the window.
- Re-launch the app by double-clicking the jar file.
Expected: The most recent window size and location is retained.
- { more test cases … }
Adding a Booking
- Adding a booking to an empty
Current Bookings
list.- Prerequisites: Have an empty
Current Bookings
list. - Test case:
add r/1 d/2023-01-01 08:00 to 2023-01-02 12:00 n/John Doe p/98765432 e/johnd@gmail.com
Expected: New booking is added.
- Prerequisites: Have an empty
- Adding a booking where there is a missing field except for
remark
field.- Prerequisites: Have an empty
Current Bookings
list. - Test case:
add r/1 d/2023-01-01 08:00 to 2023-01-02 12:00 n/John Doe p/98765432
Expected: No booking is added. Error message shown isInvalid command format! add: Adds a person to the booking book. Parameters: r/ROOM d/BOOKING PERIOD n/NAME p/PHONE e/EMAIL rk/REMARK Example: add r/1 d/2023-01-01 08:00 to 2023-01-02 12:00 n/John Doe p/98765432 e/johnd@gmail.com rk/Requested extra Queen's size bed
- Prerequisites: Have an empty
- Adding a booking where there are invalid fields.
- Prerequisites: Have an empty
Current Bookings
list. - Test case:
add r/0 d/2023-01-01 08:00 to 2023-01-02 12:00 n/John Doe p/98765432 e/johnd@gmail.com
Expected: No booking is added. Error message shown isRoom number is an integer between 1 and 500 inclusive.
- Test case:
add r/501 d/2023-01-01 08:00 to 2023-01-02 12:00 n/John Doe p/98765432 e/johnd@gmail.com
Expected: No booking is added. Error message shown isRoom number is an integer between 1 and 500 inclusive.
- Test case:
add r/1 d/2023-01-01 08:00 to 2023-01-01 08:00 n/John Doe p/98765432 e/johnd@gmail.com
Expected: No booking is added. Error message shown isBooking periods must be in the format 'YYYY-MM-DD HH:MM to YYYY-MM-DD HH:MM', and the end datetime must be after to the start datetime.
- Test case:
add r/1 d/2023-01-01 08:00 to 2023-01-02 12:00 n/John_Doe p/98765432 e/johnd@gmail.com
Expected: No booking is added. Error message shown isNames should only contain alphanumeric characters and spaces, should not be blank and should be at max 50 characters in length
- Test case:
add r/1 d/2023-01-01 08:00 to 2023-01-02 12:00 n/John Doe p/11 e/johnd@gmail.com
Expected: No booking is added. Error message shown isPhone numbers should only contain numbers, and it should be between 3 and 15 digits in length (inclusive)
- Test case:
add r/1 d/2023-01-01 08:00 to 2023-01-02 12:00 n/John Doe p/1111111111111111 e/johnd@gmail.com
Expected: No booking is added. Error message shown isPhone numbers should only contain numbers, and it should be between 3 and 15 digits in length (inclusive)
- Test case:
add r/1 d/2023-01-01 08:00 to 2023-01-02 12:00 n/John Doe p/98765432 e/johnd@notallowed.com
Expected: No booking is added. Error message shown isEmails should be of the format local-part@domain and adhere to the following constraints:
1.
The local-part should only contain at most 50 alphanumeric characters and these special characters, excluding the parentheses, (+_.-). The local-part may not start or end with any special characters.
2.
This is followed by a '@' and then a domain name. The domain name is made up of domain labels separated by periods. The domain name must end with a domain label that is supported:
-
gmail, yahoo, outlook, hotmail, icloud
- Prerequisites: Have an empty
Deleting a Booking
- Deleting a booking while all bookings are being shown
- Prerequisites: List all bookings using the
list
command. - Test case:
delete 1
Expected: First booking is deleted from the list. Message shown isDeleted Booking(s):
followed by the details of the deleted booking. For example,Deleted Booking(s): Room number: 1; Booking Period: 2023-11-08 19:28 to 2023-11-09 11:00; Name: John Doe; Phone: 98765432; Email: johnd@gmail.com; Remark: Requested extra Queen's sized bed; Room Type: NORMAL
- Test case:
delete 0
Expected: No booking is deleted. Error message shown isIndex should be a non-zero unsigned integer.
- Test case:
delete x
(where x is larger than the list size)
Expected: No booking is deleted. Error message shown isThe booking index provided is invalid
- Prerequisites: List all bookings using the
Editing a Booking
- Editing a booking while there is a non-empty
Current Bookings
.- Prerequisites: Have a fixed number of bookings currently in the
Current Bookings
list (eg: 3 Bookings) - Test case:
edit 1 p/91234567 e/johndoe@gmail.com
Expected: The first booking in the list is edited. Message shown isEdited Booking:
followed by the details of the edited booking. For example,Edited Booking: Room number: 2; Booking Period: 2023-11-08 19:28 to 2023-11-09 11:00; Name: John Doe; Phone: 91234567; Email: johndoe@gmail.com; Remark: Requested extra Queen's sized bed; Room Type: NORMAL
- Test case:
edit 0 p/91234567 e/johndoe@gmail.com
Expected: No booking is edited. Error message shown isIndex should be a non-zero unsigned integer.
- Test case:
edit 100 p/91234567 e/johndoe@gmail.com
Expected: No booking is edited. Error message shown isThe booking index provided is invalid
- Prerequisites: Have a fixed number of bookings currently in the
- Editing a booking and not changing any details in the booking.
- Prerequisites: Have an empty
Current Bookings
list, then type in the commandadd r/1 d/2023-01-01 08:00 to 2023-01-02 12:00 n/John Doe p/98765432 e/johnd@gmail.com
so that there is only 1 booking. - Test case:
edit 1
Expected: No booking is edited. Error message shown isNo changes made. At least one field to edit must be provided
- Test case:
edit 1 r/1 d/2023-01-01 08:00 to 2023-01-02 12:00 n/John Doe p/98765432 e/johnd@gmail.com
Expected: No booking is edited. Error message shown isNo changes made. At least one field to edit must be provided
- Prerequisites: Have an empty
Finding a Booking
- Finding a booking.
- Test case:
find 1
Expected: First booking is found in the list and theCurrent Bookings
only shows the first booking. Message shown is1 bookings listed!
. - Test case:
find 0
or any other find commands that do not match the name or room number of a booking.
Expected: No booking is shown. Message shown is0 bookings listed!
- Test case:
Undo the deletion of a Booking
- Undoing the deletion of a booking when a booking has been recently deleted.
- Prerequisites: delete a booking from the
Current Bookings
list. - Test case:
undo
Expected: Last deletion is undone. Message shown isLast deletion has been undone.
- Prerequisites: delete a booking from the
- Undoing the deletion of a booking when no booking has been deleted.
- Prerequisites: Have an empty
Current Bookings
by opening the application with no pre-loaded data. - Test case:
undo
Expected: No deletions is undone. Error message shown isNo deletions to undo.
- Prerequisites: Have an empty
Appendix: Effort
Appendix: Effort
Difficulty Level
- Medium
- Reason: Compared to AB3 which has one homogenous entity of Persons, CheckMate deals with 2 heterogenous entities of Persons and Bookings which adds a bit more complexity, but not as much as compared to other projects which deal with homogenous entities which have different roles that could overlap and could result in cyclical relationships (eg: Teaching Assistants and their students).
Challenges Faced
- Scope of expansion: Trying to expand AB3’s capabilities to Room Bookings was a challenge as we had to narrow down what we wanted CheckMate to do and what we could realistically make it do, for example for the Booking Periods, we could theoretically get the application to check the system’s current time and get the application to provide reminders for expiring bookings and maybe even automatically delete expired bookings, but we decided that this was out of our current scope and we would only implement it if we had the time to do so.
Effort Required
- Medium-High
- Reason: We adopted a mentality of mixing “double down or double work” when it comes to making the first iterations or skeletons of new features, which to summarise basically means that we either completely rely on the perfect implementation and on time delivery of the skeleton code by one developer (hence “double down”), or we allow the entire team to have an attempt (hence “double work”) and during the weekly team meetings we vote on which skeleton we will continue to expand upon to allow maximum creativity of the skeletons provided.
Achievements of the Project
- Expanded AB3 to the point where it looks completely new User Interface wise.
- Skillfully integrated the architecture of AB3 to work for a completely new purpose.
- Maximised the abilities of every teammate we have.
Appendix: Planned Enhancements
Appendix: Planned Enhancements
Universal Undo
- This is an enhancement to the current undo function, which currently only undoes the most recent delete command. The planned universal undo will be able to undo the most recent command, which can be any command and not just delete.
Enhanced Prefix Completion
- This is an enhancement to the current prefix completion feature which currently only loads example data when
tab
is pressed. The enhancement will instead use a trie to keep track of the most suitable next input for each field, thereby being a true prefix completion similar to autocomplete in common software such as Microsoft Word.
Room Type Allocation
- This is an enhancement to the way that room types are allocated. Currently they are allocated automatically according to the room number, however this raises an issue of having the hotel receptionist memorise which room numbers correspond to which room types. In the planned enhancement, we will allow hotel receptionists to book a room according to the room type instead of the room number, allowing them to more seamlessly make bookings without the mental overhead of memorising which rooms are which types.
Mass Add Booking
- This is an enhancement to the add command. Currently, a hotel receptionist is only able to book one room at a time, however this may be problematic when it comes to events such as weddings where one person books many rooms. In this planned enhancement, we will make the add command work similarly to the delete command which currently has a mass delete feature.